Edge list is the simplest way for graph representation. let’s say we have a graph with 5 nodes like the one below.
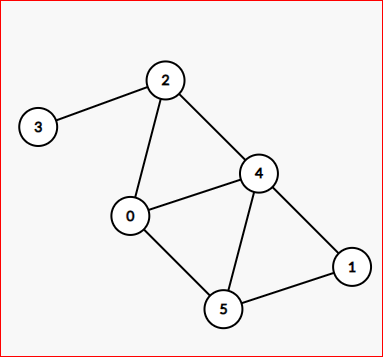
There are 5 nodes named 0, 1, 2, 3, 4, 5, and 8 edges which are (3, 2), (2, 0), (2, 4), (0, 4), (0, 5), (5,4), (4, 1), (5, 1).
In the edge list, we will make a list of pairs for edges. Each pair is an edge with starting end ending node.
Edge list in Javascript
const edgeList = [
[3,2],
[2,0],
[2,4],
[0,4],
[0,5],
[5,4],
[4,1],
[5,1]
]
Edge list in python
edgeList = [
(3,2),
(2,0),
(2,4),
(0,4),
(0,5),
(5,4),
(4,1),
(5,1)
]
Edge list in c++
#include<bits/stdc++.h>
using namespace std;
int main()
{
vector<pair<int, int> > v={
{3,2},
{2,0},
{2,4},
{0,4},
{0,5},
{5,4},
{4,1},
{5,1}
};
return 0;
}