In the realm of software development, achieving clean and maintainable code is essential to creating software systems that are scalable, robust, and long-lasting. Among the paradigms, Object-Oriented Programming (OOP) is particularly potent as it provides a systematic method for organizing code and modeling real-world scenarios. Though OOP offers a strong basis, code may quickly become too complicated to understand and maintain if best practices are not followed.
This guide teaches you how to write clean, maintainable Object-Oriented code. It covers best practices, principles, patterns, and techniques to enhance code quality and durability. It delves into strategies for readability, modularity, and technical debt, demonstrating each concept through practical examples.
Are you prepared to discover the techniques for writing code that not only satisfies modern requirements but also endures throughout time? Come along on this fascinating adventure as we reveal the secrets of maintainable, clean object-oriented code, giving you the tools you need to improve your coding skills and completely change the way you approach software development.
Understanding Clean Code Principles
Clean code is a term used to describe code that is easy to read, understand, and maintain. It was popularized by Robert Cecil Martin in his book “Clean Code: A Handbook of Agile Software Craftsmanship” in 2008. Clean code aims to create software that is not only functional but also readable, maintainable, and efficient throughout its lifecycle. It is essential for several reasons, including faster development times, improved team collaboration, easier debugging and issue resolution, and improved quality and reliability. By adhering to clean code principles, developers can create code that is not only functional but also readable, maintainable, and efficient throughout its lifecycle. By following established coding standards and writing well-structured code, developers can create higher-quality and more reliable software.
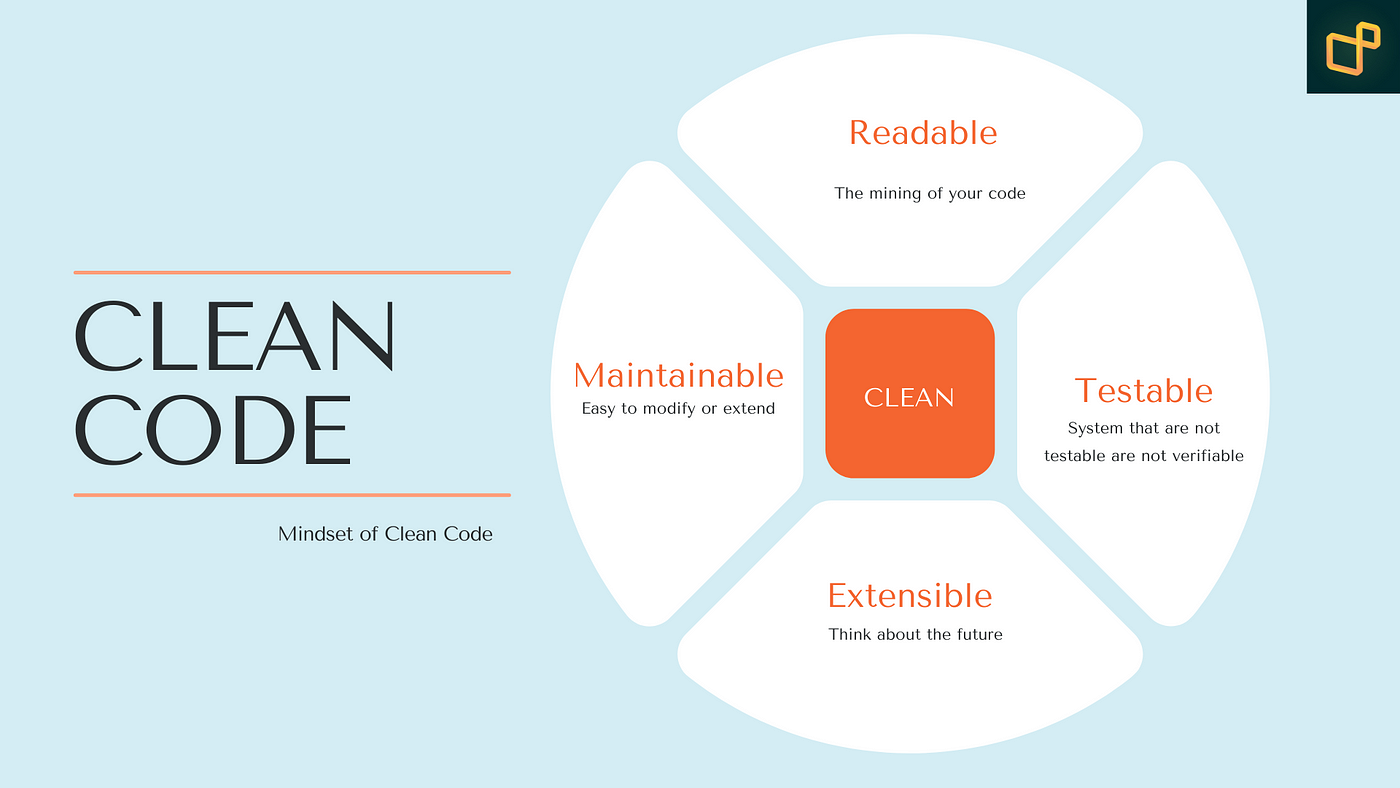
Principles of Clean Code
A well-crafted piece of code necessitates adherence to certain principles, just as a great picture requires the appropriate base and brushstrokes. These guidelines assist programmers in creating code that is easy to read, succinct, and ultimately enjoyable to work with.
- Use Meaningful and Descriptive Names: Choose names for variables, functions, and classes that reflect their purpose and behavior to make code self-documenting easier to understand without extensive comments. Robert Martin emphasizes that names should reveal the intent of the code. For example, instead of generic names like “price” and “discount,” declare variables more descriptively, like “product_price” and “discount_amount,” for better understanding.
- Follow the DRY (Don’t Repeat Yourself) Principle and Avoid Duplicating Code or Logic: Reusing code through functions, classes, modules, libraries, or abstractions improves efficiency, consistency, and maintainability. It reduces errors and bugs by requiring only one modification for changes or updates. For example, defining a single calculate_product_price function for books and laptops reduces code duplication and improves codebase maintenance.
- Comments and Documentation: Use comments sparingly and make them meaningful. Code should be self-explanatory, and documentation, like inline comments and README files, helps other developers understand purpose and usage.
- Use Meaningful Whitespace: Format code with spaces and line breaks for better readability and whitespace for logical sections, reducing cognitive load on readers.
- Error Handling: Use error-handling mechanisms like try-catch blocks to prevent crashes and provide valuable debugging information, rather than suppressing or logging errors without proper response.
- Testing: Test-driven development (TDD) encourages the creation of cleaner, well-tested code by requiring upfront consideration of edge cases and expected behavior.
Object-Oriented Design Principles
Software Design Principles are guidelines for programmers to create clear, maintainable code. Industry experts and authors recommend them. A good system design saves 20%-40% of development time, as it involves reading and maintaining the code base. A well-designed system is easy to read, understand, and extend, reducing development time and resources.
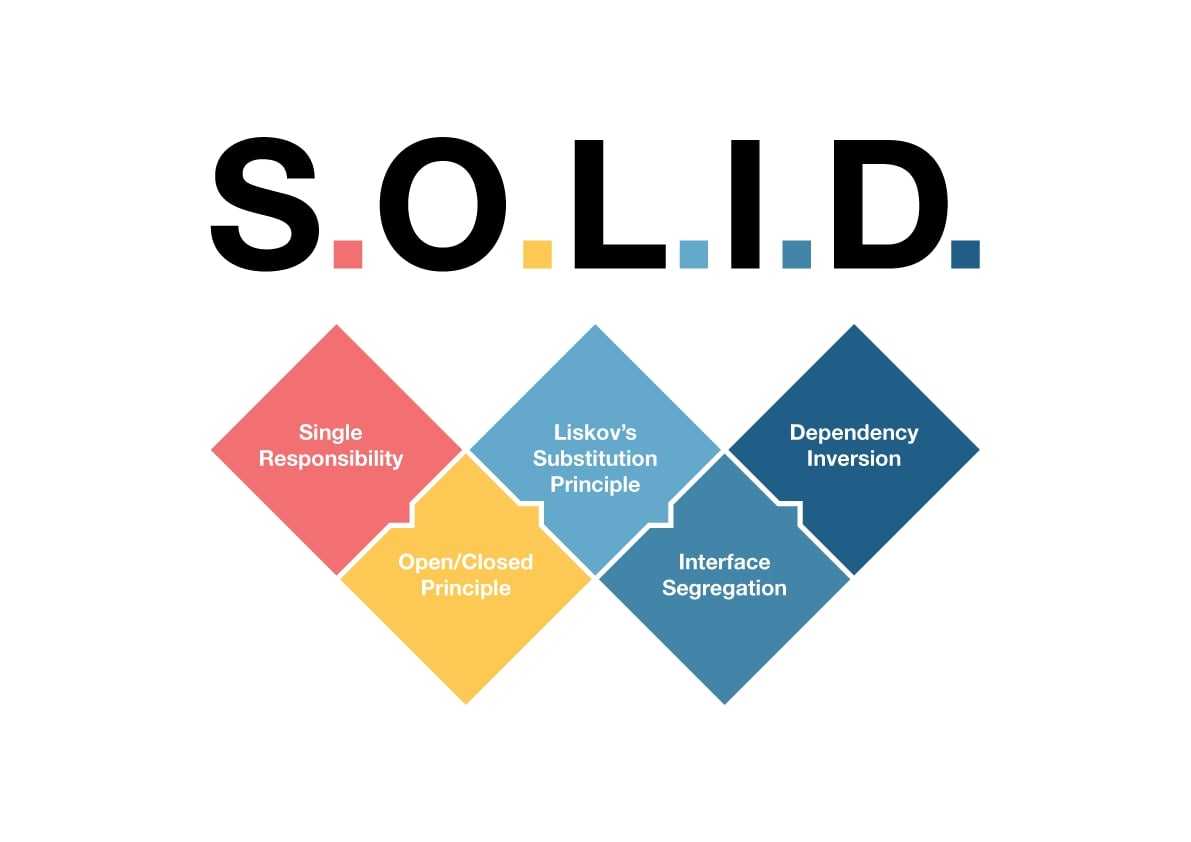
SOLID Principles
Object-Oriented Design includes these five principles:
S – Single Responsibility Principle (SRP)
O – Open-Close Principle (OCP)
L – Liskov Substitution Principle (LSP)
I – Interface Segregation Principle (ISP)
D – Dependency Inversion Principle (DIP)
These five SOLID principles are frequently used as a guide when refactoring software into a better design.
- Single Responsibility Principle (SRP): SRP (Single Responsibility Principle) is a principle in computer programming that states that every module, class, or function should have a single responsibility for a part of the program’s functionality. This principle is related to coupling and cohesion and helps classes become smaller and cleaner, making them easier to maintain. For example, an InvoiceService class with four functionalities violates SRP by putting them into a single module. Three classes should be implemented to remove this violation: InvoiceService, LoggerService, and EmailService.
- Open-Close Principle (OCP): The Open-Component-Property (OCP) principle states that software entities should be open for extension but closed for modification. To apply OCP, create new derived classes inherited from the original base class and allow the client to access the original class through an abstract interface. However, not following OCP can lead to problems, such as OCP violations when adding new logic to an application, such as adding discounts to final invoices or proposing invoices.
- Liskov Substitution Principle (LSP): The LSP is a Substitutability principle in Object-Oriented Programming (OOP), stating that objects of type T should be replaced with objects of type S if S is a subtype of T. Violations can lead to extra conditional logic and bugs, especially in shared codebases or frameworks shipped to third parties. To avoid this, use the Interface Segregation Principle (ISP) to create a new interface with only the features needed by the client code. This principle is essential in avoiding bugs and ensuring a stable and efficient application.
- Interface Segregation Principle (ISP): The ISP recommends creating numerous small interfaces based on groups of methods, with each serving one submodule. This allows clients to focus on relevant methods rather than large interfaces. For example, in a printer rental company, classes inherit the IPrinterTasks interface, which contains methods like scan or fax. To mitigate this, the interface should be split into smaller, dedicated interfaces and multiple inheritances on a class that provides additional functionality.
- Dependency Inversion Principle (DIP): DIP, the fifth SOLID principle, emphasizes that high-level modules should not depend on low-level modules, but both should depend on abstractions. It emphasizes keeping high-level and low-level modules loosely coupled to avoid breaking other classes. Other common software engineering principles include the Boy Scout Rule, Don’t Repeat Yourself, Encapsulation, the Principle of Least Astonishment, and Don’t Call Us, We’ll Call You.
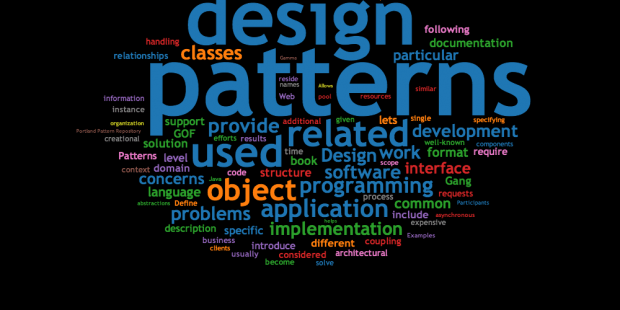
Coding Practices and Patterns
A programming paradigm known as “object-oriented design” (OOD) is centered on data structures, or “objects,” which are collections of instructions for manipulating data and data itself. When OOD is used skillfully, code may become easier to read, write, and maintain.
- Encapsulation: Encapsulation is the process of hiding the internal details of an object, revealing only necessary information. It bundles data and methods into a single unit, controlling data access and modification, reducing incorrect behavior, and enhancing data integrity.
- Inheritance: Inheritance in programming allows objects to inherit properties and behavior from another, fostering hierarchical classification. This parent-child relationship enables code reusability and polymorphism, allowing subclasses to extend or modify superclass behaviors.
- Abstraction: Abstraction simplifies complex systems by breaking them down into manageable abstract units. In OOD, abstract classes define interfaces, promoting code reusability and a clean, organized structure by defining methods without defining functionality.
- Modularity: Modularity is a system design principle that breaks down a system into smaller, functional modules, promoting the separation of concerns, and resulting in more readable, reusable, and maintainable code.
- Composition: Build more sophisticated actions out of simpler, reusable parts. To accomplish higher-level behavior, break functionality down into smaller, modular components and put them together. To encourage flexibility and ease of composition, use design patterns like composite, decorator, and strategy.
Testing and Quality Assurance
To guarantee the dependability, accuracy, and resilience of software systems, testing and quality assurance are crucial components of software development. Let’s examine many methods and approaches for quality control and testing.
Unit Testing
Testing discrete parts or code units separately to confirm their functioning is known as unit testing. For unit testing, consider the following recommended practices:
- Test Coverage: To guarantee that important components of the codebase are adequately tested, strive for high test coverage. To find regions that need more testing, use code coverage tools.
- Isolation: Make sure that external dependencies, such as file systems, databases, and network services, are segregated from unit testing. Replace external dependencies with test duplicates by using dependency injection or mocking tools.
- Test Naming: When naming unit tests, make sure the titles accurately convey the behavior under test. To enhance readability and maintainability, adhere to naming standards such as [MethodName_StateUnderTest_ExpectedBehavior].
Integration Testing
Verifying the connection and integration of various system modules or components is the main goal of integration testing. The following are some integration testing recommended practices:
- End-to-end testing: Examine the system as a whole to make sure all of its parts function as intended. Verify system behavior and mimic user interactions by using automated end-to-end testing frameworks.
- Data management: By efficiently managing test data, you may guarantee consistent and repeatable test settings. To generate test data for integration tests, employ strategies like database seeding, fixtures, or test data generators.
- Environment Isolation: To guarantee test repeatability and reliability, isolate integration tests from external dependencies. To construct isolated test environments, use methods like virtualization or containerization.
Continuous Deployment and Integration (CI/CD)
Automating the build, test, and deployment processes is a key component of continuous integration (CI) and continuous deployment (CD) procedures, which guarantee that codebase changes are verified and distributed in a timely and reliable manner. The following are some CI/CD excellent practices:
- Automated Testing: To check code changes automatically, incorporate automated tests into the CI/CD pipeline. As part of the build process, run integration tests, unit tests, and other kinds of tests.
- Incremental Builds: To reduce build times and increase developer productivity, use incremental builds. To shorten feedback times, just rebuild and test the parts that have been impacted by code modifications.
- Deployment Automation: To maintain consistency across environments and get rid of manual mistakes, automate the deployment process. Automate deployments with container orchestration systems, configuration management tools, or deployment scripts.
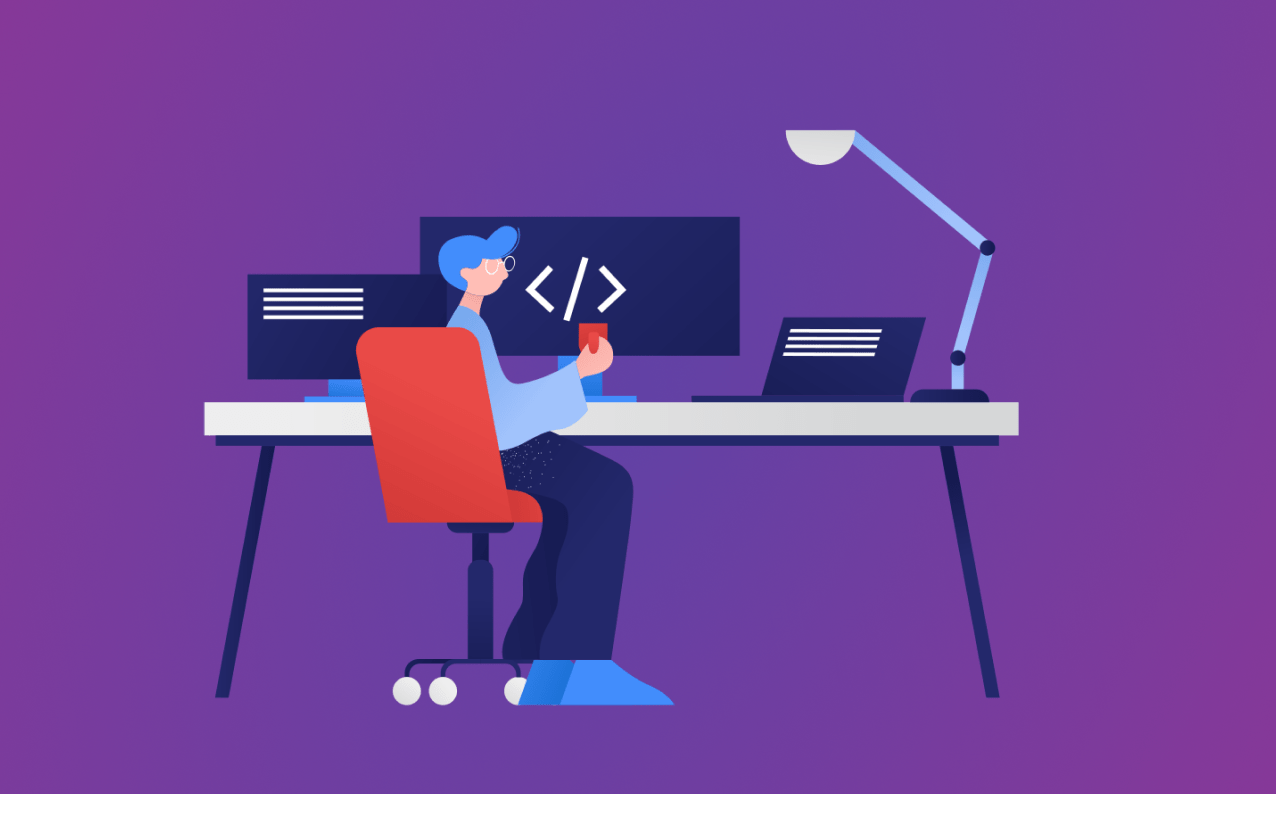
Code Refactoring and Maintenance
To guarantee a codebase’s long-term sustainability, scalability, and maintainability, refactoring and maintenance are crucial components of software development. Code refactoring is the process of reorganizing already-written code to increase readability, maintainability, and performance without affecting the code’s exterior behavior. Updating the software’s functionality, addressing technical debt, and fixing bugs are all part of maintenance. Let’s take a closer look at these subjects.
Refactoring Techniques
- Extract Method: To decrease duplication and increase readability, locate and extract complicated or repetitive code blocks into distinct methods. This method improves the modularity and readability of programming.
- Rename: To increase expressiveness and clarity, give variables, methods, and classes meaningful, descriptive names. The readability of the codebase can be greatly improved by renaming identifiers that are not well-named.
- Extract Class: Within a class, find coherent collections of behavior and data, then extract them into different classes. This method encourages better code organization and encapsulation, which facilitates understanding and maintenance.
- Replace Conditional with Polymorphism: Using inheritance or interfaces, replace conditional logic with polymorphic behavior. This method improves the code’s flexibility, extensibility, and maintainability.
- Simplify Conditionals: Break down intricate conditional statements into more manageable, easily understood terms. To cut complexity, employ strategies like ternary operators, guard clauses, and boolean algebra.
- Eliminate Dead Code: To make code more manageable and less cluttered, find and eliminate any unnecessary or outdated code. Dead code may make things more confusing for developers and make it harder for them to comprehend the system.
Best Practices for Maintenance
- Bug Fixing: To keep the program stable and dependable, prioritize and quickly resolve identified problems. To effectively find and address problems, use methodical debugging approaches including logging, unit tests, and debugging tools.
- Technical Debt Management: Identify and rank issues with code smells, design flaws, and out-of-date dependencies regularly. Every development cycle should include time and resources set aside for addressing technical debt to keep it from building up and hindering advancement.
- Enhancement Requests: Based on user input and business goals, evaluate and rank feature additions and enhancement requests. To produce value iteratively and maintain a sustainable development pace, implement new features progressively while adhering to agile development methods.
- Performance Optimization: Keep an eye on and constantly improve the system’s important code routes’ and bottlenecks’ performance. To find and fix performance problems early on, use code reviews, performance benchmarks, and profiling tools.
- Code review and version control: To efficiently work with team members and monitor changes, have an up-to-date version control system. To guarantee code quality, adherence to coding standards, and knowledge exchange among team members, conduct routine code reviews.
- Updates to the documentation: Update documentation to reflect changes made to the codebase and to give users, testers, and developers precise instructions. Keep records of design choices, architectural patterns, and coding standards to help with new team member onboarding and comprehension.
Teams may maintain the cleanliness, effectiveness, and adaptability of their codebase to changing needs by giving priority to code refactoring and maintenance tasks. Refactoring and maintenance require time and effort, but the benefits include higher code quality, increased developer productivity, and longer software maintainability.
As we conclude our investigation, it is clear that achieving clean and maintainable Object-Oriented code necessitates a comprehensive strategy that combines patterns, practices, and principles. Through the adoption of optimal practices in the areas of code organization, object-oriented design, coding approaches, testing procedures, and continuous maintenance, developers may foster codebases that are beyond ordinary utility and instead represent durability, clarity, and flexibility.
Adopting the discipline of clean code is an investment that pays off in terms of increased output, teamwork, and project sustainability. It’s not just a question of taste. The importance of clean code becomes more and more evident as software systems grow and change. It is essential for efficient teamwork, simplified development processes, and smooth code maintenance.
As we get to the end of our voyage, let’s keep in mind the lessons we’ve learned and the new perspectives we’ve gained, realizing that writing clean, maintainable object-oriented code is a continual process that requires constant progress. Let’s work together to maintain the highest standards for clean code excellence so that our software systems endure and continue to benefit users and stakeholders.