The goal of adding object-oriented capabilities to the C language led to the creation of the C++ computer language. Object-oriented programming (OOP) is a valuable tool for designers and users alike, with applications ranging from user interface design to modeling, simulation, and more. The goal of this tutorial is to provide readers with a thorough grasp of object-oriented programming in C++.
Programming was first developed with traditional methods like procedural-oriented programming, which had several drawbacks. Reusing code was difficult, access to global data created complications, and problem-solving in the actual world was less effective. However, these flaws were fixed with the introduction of object-oriented programming.
Using classes and objects, object-oriented programming makes code maintenance easier. Code reuse is made possible by inheritance, which removes the need for repetitive rewriting of the same code. Furthermore, ideas like abstraction and encapsulation make data hiding easier and improve software security and integrity.
The fundamental ideas of object-oriented programming (OOP) in C++ may be utilized by developers to produce more modular, scalable, and maintainable software. Now, let’s explore some fundamental ideas of object-oriented programming in C++ to gain a deeper understanding of its concepts and uses.
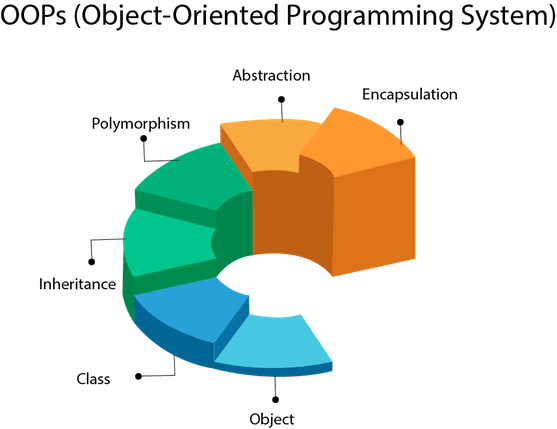
Understanding Object-Oriented Programming
Almost every developer has used object-oriented programming (OOP) at some time in their career. It is a fundamental programming paradigm. For the majority of a programmer’s academic career, OOP is taught as the normal method to code and is the most widely used programming paradigm in software development. Functional programming is another well-liked programming paradigm, although we won’t dive into it now.
We’ll cover the fundamentals of object-oriented programming today so you may begin using this paradigm with your projects, interviews, and algorithms. Let’s get started with these OOP lessons and ideas now!
The following constitute the foundation, or building blocks, of object-oriented programming:
- Classes serve as the foundation for specific objects, characteristics, and functions. They are user-defined data types.
- Instances of a class formed with specially defined data are called objects. items can be abstract entities or real-world items. The description is the sole item defined when the class is first formed.
- Functions that define an object’s actions inside a class are called methods. A reference to an instance object is the first step in any method that is specified in class declarations. In addition, instance methods refer to the subroutines that are part of an object. Programmers employ techniques to maintain functionality contained to a single object at a time or to allow for reusability.
- The state of an object is represented by its attributes, which are defined in the class template. Data about objects will be kept in the attributes field. Class properties are the property of the class.
Inheritance in C++
Inheritance is a crucial feature in Object-Oriented Programming, allowing a class to derive properties from another class. This process creates a new class called a derived class, which inherits all the properties of the base class without altering its properties. The derived class is specialized for the base class, while the base class or superclass inherits properties from the derived class.
It is feasible for classes in C++ to inherit properties and methods from one another. The “inheritance concept” is divided into two groups:
The class being inherited from is the base class (parent); the derived class (child) is the class that inherits from another class.
Use the : sign to inherit from a class.
The Car class (child) in the example below inherits the properties and methods from the Vehicle class (parent):
// Base class
class Vehicle {
public:
string brand = "Ford";
void honk() {
cout << "Tuut, tuut! \n" ;
}
};
// Derived class
class Car: public Vehicle {
public:
string model = "Mustang";
};
int main() {
Car myCar;
myCar.honk();
cout << myCar.brand + " " + myCar.model;
return 0;
}
Polymorphism in C++
“Polymorphism” is the term for having several forms. To put it simply, polymorphism is the capacity of a message to be presented in several formats. An individual who possesses many qualities at the same time is an example of polymorphism in real life. A guy is a spouse, a parent, and an employee all at once. Therefore, a person’s conduct varies depending on the circumstance. We refer to this as polymorphism. One of the key components of object-oriented programming is polymorphism.
class Enablegeeks {
public:
// Function with 1 int parameter
void func(int x)
{
cout << "value of x is " << x << endl;
}
// Function with same name but
// 1 double parameter
void func(double x)
{
cout << "value of x is " << x << endl;
}
// Function with same name and
// 2 int parameters
void func(int x, int y)
{
cout << "value of x and y is " << x << ", " << y
<< endl;
}
};
// Driver code
int main()
{
Enablegeeks obj1;
// Function being called depends
// on the parameters passed
// func() is called with int value
obj1.func(7);
// func() is called with double value
obj1.func(9.132);
// func() is called with 2 int values
obj1.func(85, 64);
return 0;
}
Abstraction in C++
Data abstraction is a crucial feature of object-oriented programming in C++, displaying only essential information while hiding background details or implementation. It’s like a man driving a car, unaware of the inner mechanism or implementation of the car’s functions. This approach helps in understanding the data’s functionality.
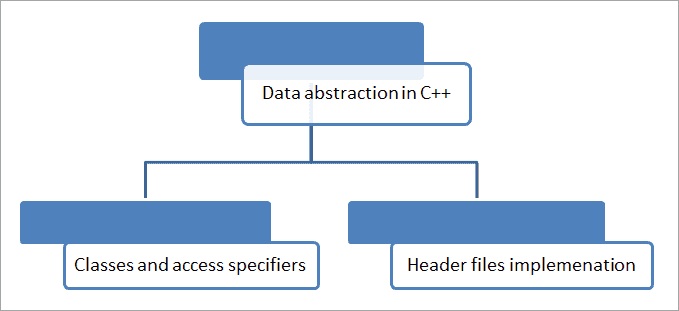
#include<iostream>
using namespace std;
class Vehicle
{
private:
void piston()
{
cout<<"4 piston\n";
}
void manWhoMade()
{
cout<<"Markus Librette\n";
}
public:
void company()
{
cout<<"GFG\n";
}
void model()
{
cout<<"SIMPLE\n";
}
void color()
{
cout<<"Red/GREEN/Silver\n";
}
void cost()
{
cout<<"Rs. 60000 to 900000\n";
}
void oil()
{
cout<<"PETRO\n";
}
};
int main()
{
Vehicle obj;
obj.company();
obj.model();
obj.color();
obj.cost();
obj.oil();
}
Advanced OOP Techniques
More complex C++ Object-Oriented Programming (OOP) approaches let programmers write more effective, adaptable, and maintainable code. Let’s examine some of these sophisticated methods in more detail:
Templates and Generic Programming
C++ templates offer a robust generic programming approach that enables programmers to create code that functions with any data. Important features of templates include:
- Function Templates: Create functions that don’t require duplicate code to work on different forms of data. Function templates are very handy for constructing general algorithms like searching and sorting.
- Class Templates: Make classes that can have one or more data types parameterized. Generic data structures, algorithms, and container classes can be created with the help of class templates.
- Template metaprogramming: Make use of templates’ compile-time evaluation features to carry out intricate calculations and produce dynamic code. Compile-time optimizations and the implementation of static polymorphism are common uses for template metaprogramming.
Standard Template Library (STL)
The C++ Standard Library provides a set of generic algorithms, containers, and utilities that make up the Standard Template Library (STL). Important elements of the STL consist of:
- Containers: Effective data structures for storing and modifying groups of objects are provided by STL containers like vectors, lists, maps, and sets. The general nature of containers and their smooth integration with STL algorithms are built in.
- Iterators: Without relying on the underlying data structure, algorithms may operate on container items thanks to STL iterators, which offer a consistent interface for traversing elements in containers.
- Algorithms: A variety of generic algorithms are available for manipulating container items using STL algorithms including sorting, searching, and reshaping. Algorithms may be applied to any STL-compatible container and are meant to be used with iterators.
Smart Pointers and Memory Management
Smart pointers are C++ objects that offer intelligent memory management and behave similarly to raw pointers. Typical smart pointer types consist of:
- A dynamically created object’s unique_ptr indicates exclusive ownership and makes sure the object is removed when the unique_ptr is no longer in use.
- To control shared ownership of a dynamically created object, shared_ptr uses reference counting. The item is removed when the last shared_ptr that owned it is destroyed.
- weak_ptr: Gives a shared object non-intrusive access without extending its lifetime. If the object is still there, a weak_ptr can be changed to a shared_ptr.
In C++ programs, smart pointers make memory management easier and help avoid frequent problems like memory leaks and dangling pointers.
Advanced Inheritance and Polymorphism
OOP’s core ideas of inheritance and polymorphism may be implemented with ease because of C++’s sophisticated features:
- Virtual Inheritance: Use virtual inheritance to clear up any confusion caused by multiple inheritance. In the derived class hierarchy, virtual inheritance guarantees that a base class is represented by a single instance.
- Final and Override Specifiers: To clearly state that a member function is meant to replace a virtual function from a base class, use the override specifier. To stop additional virtual function overriding in derived classes, use the final specifier.
- Abstract Base Classes: To establish interfaces and mandate polymorphic behavior in derived classes, define abstract base classes using only virtual functions. Although they cannot be directly instantiated, abstract base classes can be used to establish shared interfaces for classes that are related.
Template Metaprogramming
Using the capability of C++ templates, template metaprogramming generates code at compile time and performs calculations. Important methods in template metaprogramming consist of:
- Compile-Time Computations: Utilize templates to carry out compile-time operations including conditional branching, type manipulation, and arithmetic operations. Code generation may be customized, optimizations can be carried out, and constant values can be generated via compile-time calculations.
- Template Specialization: To offer tailored behavior or optimizations, specialize templates for particular data kinds or situations. With template specialization, developers may enhance performance and customize template implementations to meet particular needs.
- Utilize the SFINAE (Substitution Failure Is Not An Error) concept to manage template instantiation and allow or prohibit template overloads according to type characteristics or requirements. SFINAE makes it possible to create generic code that can adapt to many conditions and to create more adaptable template designs.
Developers may write more expressive, effective, and reusable code and enable the creation of scalable and reliable software by learning these advanced OOP concepts in C++. By using these strategies, developers may fully utilize the C++ language and take on challenging programming tasks.
Best Practices and Design Patterns
For C++ object-oriented programming (OOP) code to be effective, maintainable, and scalable, best practices and design patterns are essential. Let’s examine a few of the most significant routines and trends:
Design Patterns in C++
Reusable solutions to typical design issues that arise throughout software development are offered by design patterns. They contain tried-and-true methods for resolving particular design issues. Among the essential C++ design patterns are the following:
- Singleton Pattern: A class with a single instance and a global point of access to it are guaranteed by the singleton pattern. Classes that have a single common state that they must keep throughout the application can benefit from the Singleton design.
- Factory Pattern: The factory pattern specifies an interface for object creation, but it gives subclasses the ability to change the kind of object that is generated. Decoupling object generation from client code and facilitating polymorphic object development are two benefits of the Factory pattern.
- Observer Pattern: Describes a one-to-many dependence between objects in which any changes in the subject’s state are reported to its observers. When developing event-driven systems and preserving loose connectivity between components, the Observer pattern comes in handy.
- Strategy Pattern: A strategy pattern is a set of algorithms that are defined, encapsulated, and interchangeable. The Strategy pattern makes runtime algorithm selection easier and lets algorithms change regardless of the clients using them.
- Decorator Pattern: The decorator pattern dynamically adds further responsibilities to an object. As an adaptable substitute for subclassing, decorators allow you to increase an object’s capabilities at runtime.
Error Handling and Exception Safety
A crucial component of developing software is handling failures, and C++ offers several methods for doing so while maintaining exception safety:
- Exception Handling: To handle runtime problems gracefully, use exception-handling methods like try-catch blocks and throw statements. A way to propagate mistakes across function boundaries and unwind the call stack to an appropriate error-handling context is through exceptions.
- RAII (Resource Acquisition Is Initialization): Use the RAII (Resource Acquisition Is Initialization) idiom to make sure that resource management and exception safety are done correctly. RAII ensures that resources are correctly cleaned up even in the case of exceptions by obtaining resources (such as memory, file handles, and locks) in constructors and releasing them in destructors.
- No-Throw Guarantee: To guarantee consistent behavior and uphold exception safety, wherever feasible, design functions and APIs with a no-throw guarantee. Functions that promise not to throw exceptions can be used in crucial parts of the code when it’s important to reduce the cost associated with exception handling.
Testing and Debugging Practices
In software development, testing and debugging are crucial tasks that guarantee the accuracy, dependability, and resilience of C++ code:
- Unit Testing: To verify the operation and behavior of distinct classes, functions, and components, write unit tests for them. Automating testing and confirming that code complies with requirements and specifications is made possible by unit tests.
- Integrity testing: Integrity testing is done to verify how various application modules, subsystems, and components interact with one another. Compatibility problems, interface inconsistencies, and inconsistent behavior may all be found with the use of integration tests.
- Code Reviews: Examine C++ code for accuracy, quality, and maintainability by conducting code reviews. Code reviews entail a methodical inspection of the code for mistakes, defects, poor design, and compliance with best practices and standards.
Performance Optimization
Performance optimization in C++ code is a continuous process that includes finding and fixing resource utilization problems, bottlenecks, and inefficiencies:
- Profiling: To determine areas for optimization, examine the performance characteristics of C++ programs using profiling tools. Performance-affecting hotspots, memory leaks, and CPU-intensive tasks may all be found with the use of profiling.
- Algorithmic Optimization: To increase the effectiveness of C++ programs, optimize data structures and algorithms. Select data structures and methods that reduce computing complexity and resource consumption and are appropriate for the issue domain.
- Compiler Optimization: To enhance the efficiency of C++ code, activate compiler optimizations. Inlining, loop unrolling, instruction scheduling, and other methods the compiler uses to generate code are examples of compiler optimizations.
These best practices can help developers construct C++ codebases that are reliable, efficient, and match the needs of modern software development. They can also help developers use design patterns efficiently. By using these techniques, programmers may create software solutions that are simpler to comprehend, expand, and maintain over time. They can also produce cleaner, more expressive code.
To sum up, C++’s Object-Oriented Programming (OOP) is a fundamental component of software development, providing a flexible and strong method for creating solutions. Developers may produce software that is effective, manageable, and scalable, meeting the changing requirements of contemporary applications, by adopting the concepts and techniques of object-oriented programming (OOP).
Programming languages still rely heavily on C++ because of its robust feature set, large library, and strong community support. Whether creating complex business solutions, visual user interfaces, or engaging games, OOP in C++ gives developers the skills and tools they need to succeed in the cutthroat world of modern software development.
Developers may unleash a world of possibilities and push the frontiers of creativity while producing high-quality software solutions that endure over time by learning OOP in C++. C++ is still a top option for anyone looking to use Object-Oriented Programming in their projects because of its dependability and adaptability.