Procedural programming, a paradigm centered on procedure calls—each procedure consisting of a series of computing steps—is the foundation of traditional programming languages like C, FORTRAN, and Pascal. Programmers find procedural programming to be very intuitive and approachable, which makes it a popular choice. But maintaining and changing code gets more and harder as codebases get bigger and more complex. Something as simple as changing a single function might set off a chain reaction of errors that result in “spaghetti code.”
Computer architects created object-oriented programming (OOP) and functional programming as more modern paradigms to counter the drawbacks of procedural programming. These paradigms provide several ways to organize code with the goals of improving robustness, scalability, and maintainability.
Every paradigm offers a unique approach to managing and organizing code. We’ll examine the foundational ideas of functional and object-oriented programming in this post and their advantages, applications, and use cases in contemporary software development. Comprehending these programming paradigms is crucial for navigating the always-changing field of programming, whether large-scale software project complexity or code efficiency optimization.
Understanding Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a computer science paradigm that uses classes and objects to structure software programs into reusable code blueprints. Common OOP languages include JavaScript, C++, Java, and Python. Classes are abstract blueprints that create concrete objects, representing broad categories with shared attributes. They can also contain methods that perform specific actions for specific object types.
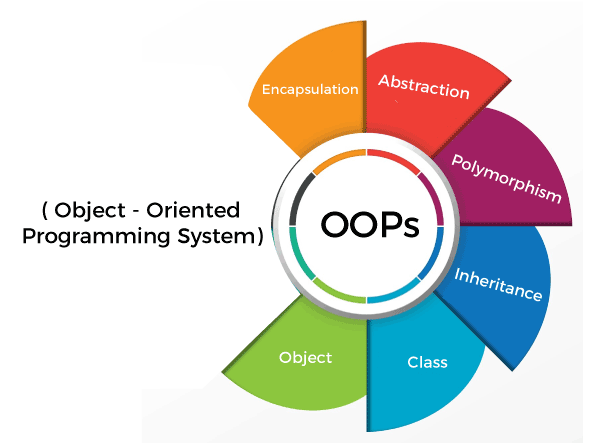
Characteristics of OOP
Object-oriented programming (OOP) is a programming paradigm that emphasizes the use of objects to define business rules, exchange data, and control the programming environment. It involves creating a blueprint for objects, known as the interface, which specifies their methods, properties, and other aspects. The interface is not static and can be changed by programmers to account for new interactions.
OOP also emphasizes the encapsulation of data and functionality within objects, which prevents hackers from modifying stored data. Encapsulation and abstraction of data are crucial components of OOP, improving security and scalability in programming frameworks.
Abstraction is the process of creating reusable objects that standardize common business logic. It encourages collaboration between different parties, making it easier to integrate disparate data sets and functionality. Classes and Objects are the next characteristic of OOP, where the blueprint for an object is defined, and objects are created from classes.
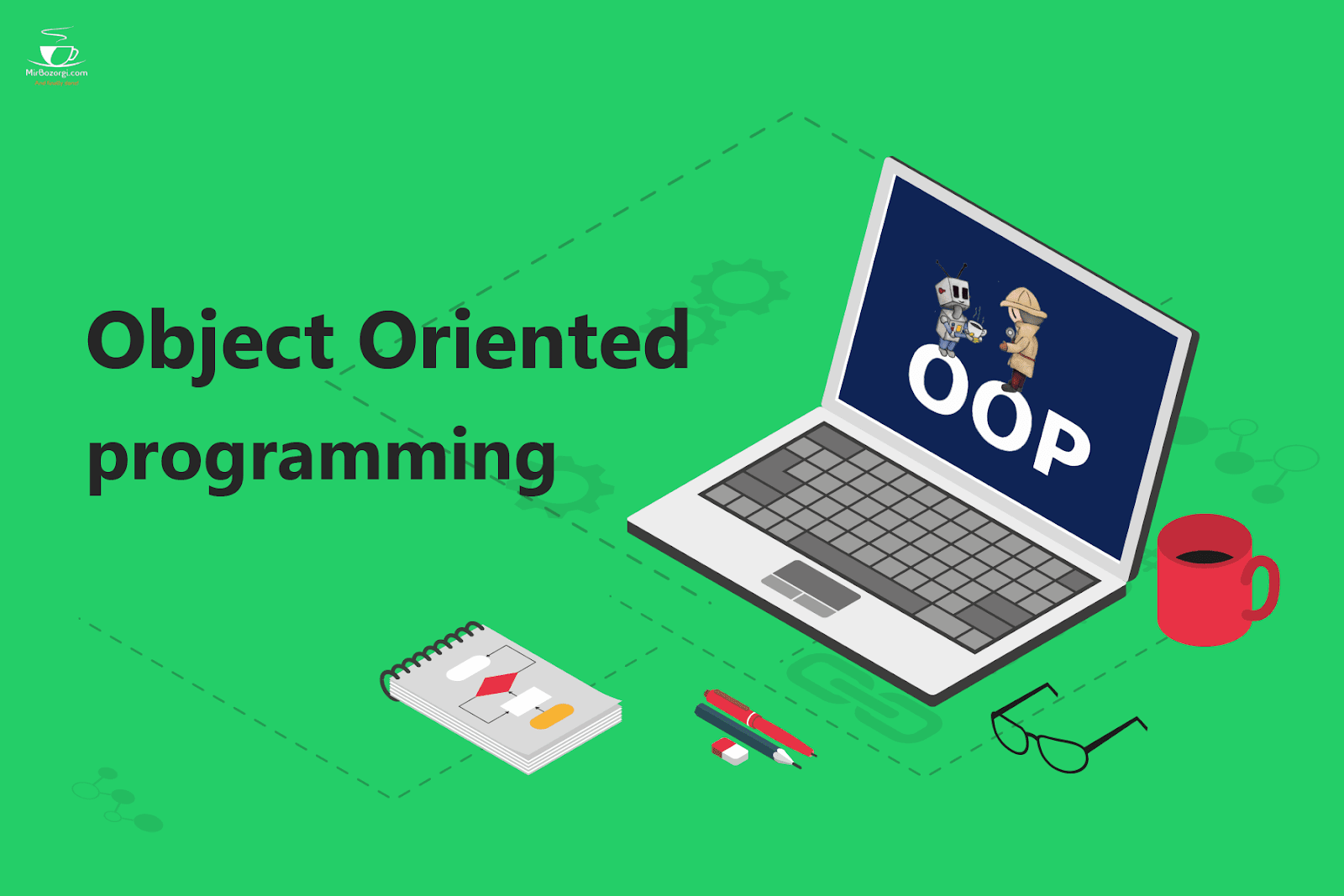
OOP is a programming paradigm that focuses on inheritance and composition, which allow one object to create another. Composition involves combining multiple objects to create a new, unique object. Inheritance and composition are crucial aspects of OOP, as they allow programmers to reuse specific elements from other objects.
Binding is another important aspect of OOP, linking properties of an object to another in an application. There are two types: dynamic binding, which changes the value of another property, and static binding, which sets one property without changing another. Dynamic binding is more difficult and can lead to errors.
Message passing is another crucial aspect of OOP, allowing objects to communicate with each other and build complex chains of objects. Objects can send messages from one object to another, allowing the entire program to be made up of multiple objects communicating with each other. This makes it easier for programs to become more complex and for objects to interact in interesting ways.
OOP is popular for its better code quality, increased productivity, maintainability, scalability, and ease of understanding. It is also popular due to the need for seamless transitions between desktop and mobile platforms, interactive interfaces, and data-driven decisions.
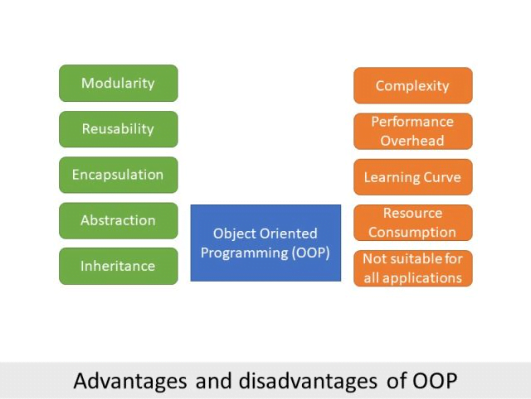
Strengths and Weaknesses of OOP
OOP (Object-Oriented Programming) is a framework that allows code reuse and modularity by defining classes and inheriting common attributes from parent classes. This reduces the amount of code needed and makes it easier to modify and extend existing code. Encapsulation, a method of grouping related data and functions, improves readability and security. However, OOP can increase code complexity and size, making it harder to understand, debug, and test. Additionally, it consumes more memory and CPU resources than other paradigms like procedural or functional programming.
Exploring Functional Programming (FP)
The paradigm of software development is revolutionized by Functional Programming (FP), and Go is a contemporary language that gracefully supports this idea. As in classic object-oriented languages, functions are regarded as first-class citizens in FP. This idea creates a whole new range of program structure options. Let’s explore this idea with a special Go example. Let’s examine the fundamental ideas and characteristics of functional programming in more detail:
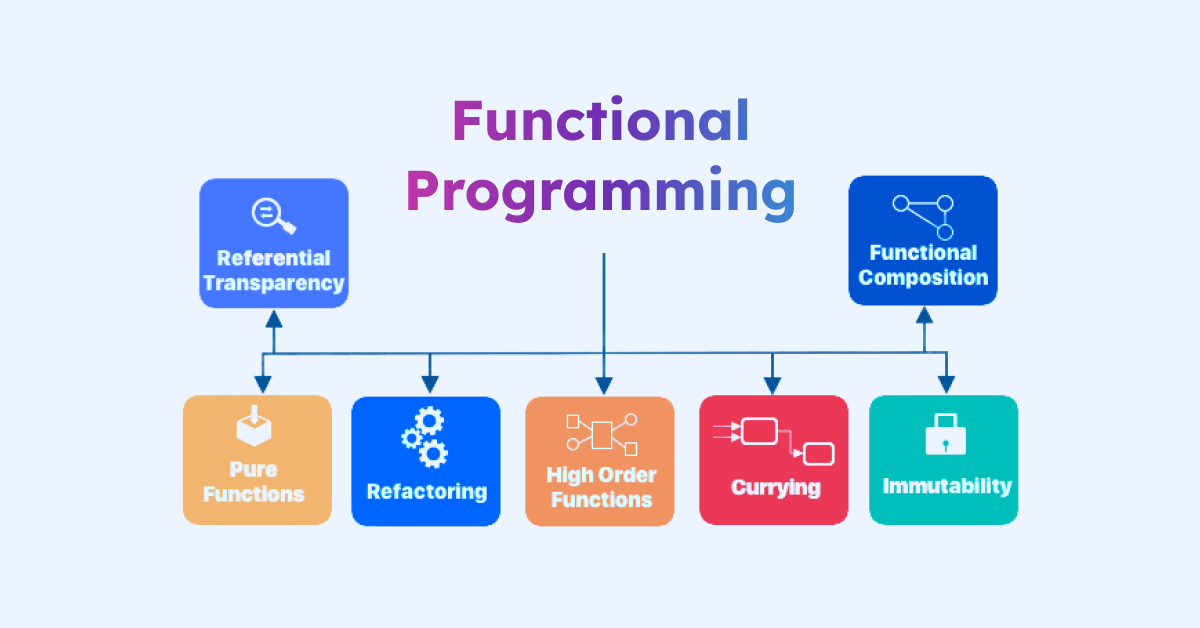
- Pure Functions: Pure functions in Functional Programming are the fundamental paradigm, ensuring consistent output without any external state changes. They are deterministic and transparent, making them easier to reason about and test.
- Immutability: Functional Programming emphasizes immutable data structures, which prevent modifications after creation. This approach addresses shared mutable state and concurrency issues by keeping each piece of data isolated.
- Higher-Order Functions: Functional Programming heavily uses higher-order functions like map, filter, reduce, and compose to abstract common patterns and behaviors, enabling developers to write concise, expressive code.
- Function Composition: Function composition is a fundamental concept in Functional Programming, combining multiple functions to create new ones, enabling developers to build complex behavior in a declarative, expressive style.
- Declarative Programming: Functional Programming emphasizes a declarative style, describing desired outcomes rather than step-by-step instructions. Declarative code is more concise, readable, and maintainable than imperative code.
- Immutable Data Structures: Functional programming languages support immutable data structures like lists, sets, maps, and tuples, enabling thread-safe, race-free code and encouraging functional-style programming through data manipulation and transformation.
- Lazy Evaluation: Lazy evaluation is a technique in Functional Programming languages that delays expression evaluation until needed, enabling efficient resource use and expressive programming in infinite data structures.
Functional Programming, a distinct approach to software development, uses pure functions, immutability, and declarative programming to create predictable, maintainable, and scalable code. Languages like Haskell, Clojure, and Scala offer powerful tools for expressing complex ideas.
Advantages and disadvantages of functional programming
Advantages:
- Simple: Functional programming (FP) is a structured approach to writing code that emphasizes simplicity, clarity, and conciseness. Pure functions and immutable variables provide a structure that helps write simple code, as they isolate data and pure functions from side effects and mutations, reducing error handling and null checks. FP also uses declarative code, which describes intent and is simple. Understanding the advantages and disadvantages of FP is crucial before fully embracing it, and this blog post aims to help readers decide if FP is a suitable solution for their problem.
- Testable: Pure functions don’t require mocks for testing, allowing the entire application core to be tested without mocks in FP. This method allows mathematical proof of the correctness of pure functions, making unit tests easier and faster, providing high confidence in implementation correctness.
- Reusable: FP has three properties that make its functions highly reusable: First Class Functions, which allow functions to be passed as parameters and return functions, and Composition, which allows functions to be chained and combined. Currying and partial application are techniques used to reuse the logic of a function in different contexts, similar to method overloading in other languages. These properties enable functions to be reused in various scenarios.
- Scalable: Modern CPUs have numerous cores, necessitating software to handle threads and concurrency. Pure functions and immutable data make programs thread-safe, enabling scalability.
Disadvantages:
- Memory usage and performance: Immutable variables create new variables, doubling memory usage and impacting performance in large, nested data structures. Functional languages like tail call optimization, lazy evaluation, and smart linking help avoid this trade-off, but multi-paradigm or OOP languages may not have these optimizations
- Fewer frameworks and tools: Functional programming languages like Java and JavaScript have a larger community, leading to more frameworks, tools, and reusable packages. However, in the age of cloud and containers, language support restrictions persist in various cloud services and tools.
- Fewer users/experts: When adopting a functional programming language, companies must consider the availability of experts in the language, as sourcing from a specialized vendor may be more expensive due to language scarcity.
Choosing the Right Paradigm
The development process, maintainability, and scalability of the product may all be greatly impacted by choosing the right programming paradigm for a project. Two different methods of problem resolution and code organization are provided by Object-Oriented Programming (OOP) and Functional Programming (FP). Let’s take a closer look at the factors to consider while selecting the appropriate paradigm:
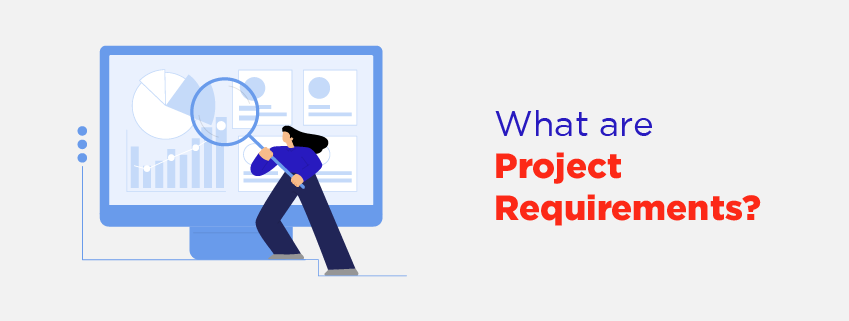
Understand Project Requirements
Comprehending the project’s requirements and limitations in detail is crucial before selecting a programming paradigm. Take into account elements like the project schedule, team experience, scalability requirements, performance requirements, and issue domain nature.
- Performance Requirements: The choice of paradigms, such as OOP or FP, can significantly impact performance in high-performance computing or real-time systems, with OOP offering better performance due to mutable state and imperative control flow.
- Complexity of the Problem Domain: OOP is ideal for modeling real-world entities and relationships, while FP offers concise, expressive solutions for data transformation and manipulation.
- Scalability Needs: The project’s scalability requirements are considering two paradigms: OOP’s encapsulation-based approach, which can cause complex synchronization issues, and FP’s immutable data-based approach, which simplifies concurrency management.
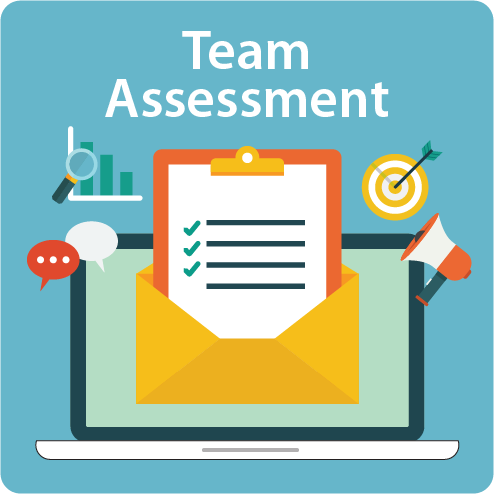
Assess Team Expertise and Experience
The development team’s expertise and experience in OOP and FP concepts, languages, and tools are crucial when selecting a programming paradigm.
- OOP Proficiency: The team with extensive OOP experience can effectively utilize OOP principles and tools for the project, accelerating development and reducing the learning curve.
- FP Proficiency: The team, with experience in functional languages like Haskell, Scala, or Clojure, may consider adopting an FP approach for concise, expressive, and maintainable solutions.
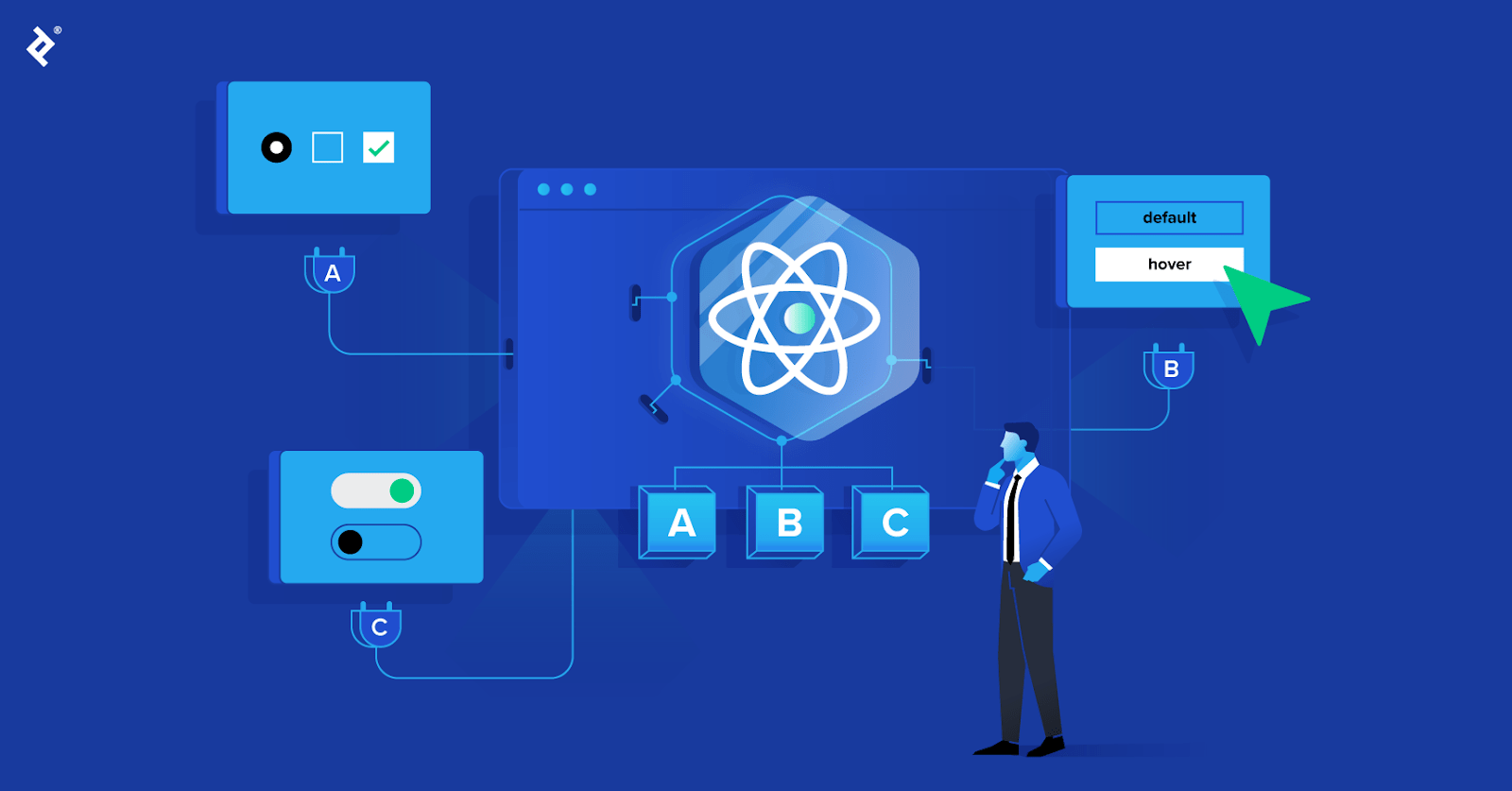
Consider Tooling and Ecosystem
Analyze each programming paradigm’s accessibility to tools, libraries, frameworks, and community support. Take into account elements like community involvement, library accessibility, ecosystem maturity, and language popularity.
- OOP Ecosystem: OOP languages like Java, C#, and Python offer robust ecosystems with extensive libraries, frameworks, and tools, facilitated by large developer communities and industry support.
- FP Ecosystem: FP languages, like Haskell, Scala, and Clojure, offer expressive type systems, powerful abstractions, and functional libraries, despite smaller communities and resources compared to mainstream OOP languages.
Evaluate Trade-offs
Think about the tradeoffs and trade-offs that come with each programming paradigm. There are advantages and disadvantages to both OOP and FP, and selecting a paradigm requires weighing trade-offs in light of the demands and limitations of the project.
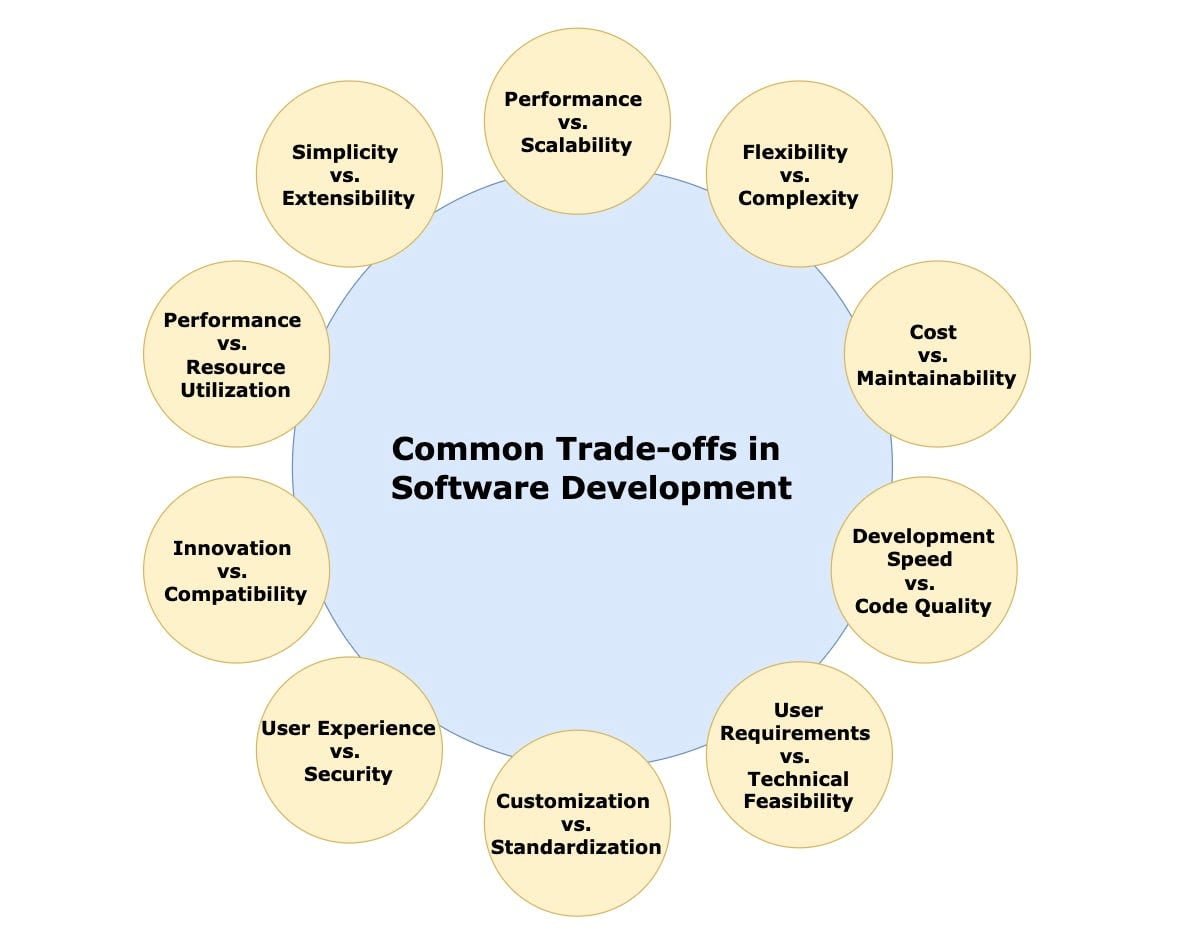
- OOP Trade-offs: OOP is adept at modeling complex systems with rich domain models, but can lead to tight coupling, mutable state, and inheritance hierarchies, making code harder to understand and maintain.
- FP Trade-offs: FP, a programming language that emphasizes immutable data, pure functions, and higher-order abstractions, can be challenging for developers accustomed to imperative programming and may not suit all problem domains.
Consider Hybrid Approaches
A hybrid strategy that incorporates aspects of both OOP and FP could be the best option in some circumstances. The OOP and FP paradigms are supported by a wide range of contemporary programming languages, including Scala, Kotlin, and TypeScript, enabling programmers to take use of each paradigm’s advantages as needed.
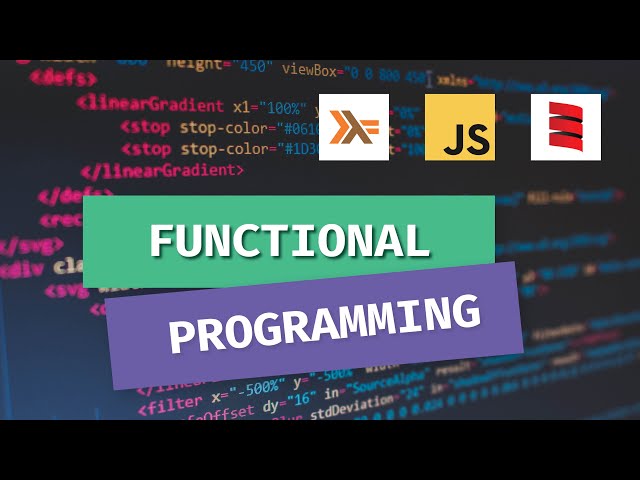
- Scala: Scala seamlessly integrates OOP and FP features, enabling developers to write code in imperative or functional styles, thanks to its type system, pattern matching, and higher-order functions.
- Kotlin: Kotlin, a popular choice for Android development and backend services due to its concise syntax and compatibility with Java libraries, supports OOP and FP concepts.
- TypeScript: TypeScript, a superset of JavaScript, enhances maintainability and functionality in large-scale codebases by adding static typing, classes, and interfaces.
Selecting the right programming paradigm for a project requires careful consideration of project requirements, team expertise, ecosystem support, and trade-offs. Understanding OOP and FP strengths and weaknesses helps developers make informed decisions for robust, maintainable, and scalable software solutions.
As we conclude our examination of Object-Oriented Programming (OOP) and Functional Programming (FP), it is important to understand that there is no one-size-fits-all approach to choose between these two paradigms. Rather, it depends on the particular specifications, limitations, and applications of your project.
You may choose a paradigm that best suits your project’s objectives and your development team’s experience by carefully examining the tenets, traits, and trade-offs of each one.
Do you like OOP’s modularity and flexibility, which allow for the reuse and maintainability of code by encapsulating data and action in objects? Or maybe the simplicity and parallelism of FP—where functions are seen as first-class citizens and immutable data structures are the norm—have drawn you in?
The secret is to comprehend the subtleties of your project and identify the paradigm that best meets its requirements. In the ever-changing world of contemporary software development, both the OOP and FP paradigms provide strong tools for designing resilient, scalable, and maintainable software systems.
In order to create software solutions that last over time, take into account the distinctive qualities of each paradigm as you begin your programming adventure and take advantage of the chance to play to their advantages.