Java programming has two classes that offer methods for constructing and modifying strings: StringBuilder and StringBuffer. Despite their shared functions, there are important distinctions between the two that may impact consumption and performance in certain situations. We’ll go into the nuances of StringBuilder and StringBuffer in this extensive guide, examining their parallels, differences, and optimal usage scenarios.
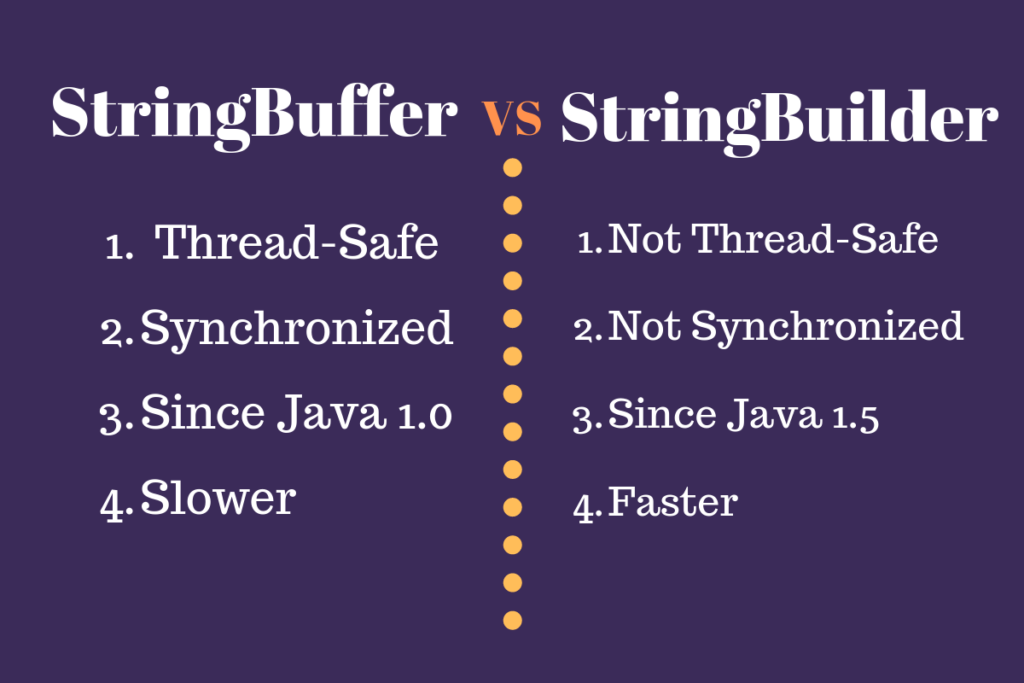
Java classes StringBuilder and StringBuffer both offer modifiable character sequences. This implies that you don’t have to create a new object every time you want to change the contents of a StringBuilder or StringBuffer object. When you need to concatenate several strings or conduct other string manipulation tasks, this can be helpful.
String in Java
In Java, strings are internal objects that have a char array backing them up. Strings are immutable because arrays are immutable (they cannot expand). A whole new String is produced whenever a String is modified. Nonetheless, strings may be used through a variety of classes that Java offers. StringBuffer and StringBuilder are two examples of such classes. We shall examine the distinctions between the two classes in this post.
StringBuilder
In Java 5, StringBuilder made its debut as a component of java.lang package. It is not suited for usage in multi-threaded applications because many threads may access the same object concurrently since it is not thread-safe. Because StringBuilder does not synchronize, it performs better than StringBuffer in single-threaded applications.
StringBuffer
Since the early days of Java, StringBuffer has existed as a heritage class. Its functionality is identical to StringBuilder’s, but it is safe to use in multi-threaded programs without compromising data integrity since it is thread-safe. StringBuffer achieves thread safety by synchronizing access to its methods, which in some cases might affect speed.
Comparison of StringBuilder and StringBuffer
Mutability
Both StringBuilder and StringBuffer offer character sequences that are changeable, so you can change the contents of the string without having to make a new one.
Performance
Because StringBuilder is not synchronized, it often performs better than StringBuffer in single-threaded applications. Faster execution speeds may result from this absence of synchronization overhead, particularly in scenarios where string manipulation operations are regularly carried out.
Thread Safety
Because StringBuffer is thread-safe, there is no chance of data corruption while using it in multi-threaded applications. However, because StringBuilder lacks thread safety, it should not be used in situations where many threads may be concurrently accessing the same object.
Synchronization
By synchronizing access to its operations, StringBuffer makes sure that only one thread may access the object at once and provides thread safety. Performance may be impacted by this synchronization cost when several threads are concurrently accessing the object.
From String to StringBuffer and StringBuilder
This is a simple workaround as the constructors of the StringBuffer and StringBuilder classes may receive the String class object directly. Since the Java String class is immutable, we can alter a string by converting it to an object of the StringBuffer or StringBuilder classes.
public class EG{
public static void main(String[] args)
{
// Custom input string
String str = "Enable";
// Converting String object to StringBuffer object
// by
// creating object of StringBuffer class
StringBuffer sbr = new StringBuffer(str);
// Reversing the string
sbr.reverse();
// Printing the reversed string
System.out.println(sbr);
// Converting String object to StringBuilder object
StringBuilder sbl = new StringBuilder(str);
// Adding it to string using append() method
sbl.append("Geek");
// Print and display the above appended string
System.out.println(sbl);
}
}
StringBuilder to StringBuffer Conversion
It is not possible to convert StringBuffer to StringBuilder directly. Using the built-in toString() function, we must first convert the StringBuffer to a String object. We can easily create a StringBuilder using the class constructor after converting it to a string object. As an illustration:
public class EG {
public static void main(String[] args)
{
// Custom input string
String str = "Enable";
// Converting String object to StringBuffer object
// by
// creating object of StringBuffer class
StringBuffer sbr = new StringBuffer(str);
// Reversing the string
sbr.reverse();
// Printing the reversed string
System.out.println(sbr);
// Converting String object to StringBuilder object
StringBuilder sbl = new StringBuilder(str);
// Adding it to string using append() method
sbl.append("Geek");
// Print and display the above appended string
System.out.println(sbl);
}
}
From StringBuffer and StringBuilder to String
The toString() function, which is overridden in the StringBuffer and StringBuilder classes, can be used to carry out this translation. The Java application to illustrate the same is shown below. The character sequence that the StringBuffer object presently represents is initialized and allocated to a new String object (in the Heap region) when we use the toString() function. This implies that modifications made to the StringBuffer object in the future will not impact the contents of the String object.
public class EG {
public static void main(String[] args)
{
// Creating objects of StringBuffer class
StringBuffer sbr = new StringBuffer("Enable");
StringBuilder sbdr = new StringBuilder("Hello");
// Converting StringBuffer object to String
// using toString() method
String str = sbr.toString();
// Printing the above string
System.out.println(
"StringBuffer object to String : ");
System.out.println(str);
// Converting StringBuilder object to String
String str1 = sbdr.toString();
// Printing the above string
System.out.println(
"StringBuilder object to String : ");
System.out.println(str1);
// Changing StringBuffer object sbr
// but String object(str) doesn't change
sbr.append("Geek");
// Printing the above two strings on console
System.out.println(sbr);
System.out.println(str);
}
}
Performance Considerations
A key factor to keep in mind while deciding between StringBuilder and StringBuffer is how each class will perform. Due to its lack of synchronization overhead, StringBuilder often performs better in single-threaded settings where thread safety is not an issue. Nevertheless, despite its possible impact on speed, StringBuffer could be the better option in multi-threaded applications where thread safety is crucial.
Thread Safety
Thread safety is one of the main distinctions between StringBuilder and StringBuffer. Because StringBuffer is intrinsically thread-safe, data corruption is not possible when using it in multi-threaded applications. However, as StringBuilder lacks thread safety, it shouldn’t be employed in situations involving several threads that could access the same item at once.
StringBuilder vs. StringBuffer Decision
Consider the following elements while choosing between StringBuilder and StringBuffer:
- Thread Safety: Use StringBuffer if thread safety is required for your application. You can use StringBuilder for faster efficiency if thread safety is not an issue.
- Performance: Because StringBuilder doesn’t have synchronization overhead, it usually performs faster in single-threaded settings. Despite its influence on speed, StringBuffer could be a superior option for multi-threaded applications.
We have examined the differences between Java’s StringBuilder and StringBuffer in this guide. While both classes provide changeable character sequences, there are differences between them in terms of synchronization, thread safety, and efficiency. Understanding these differences and assessing the requirements of your application will help you choose the best class for the string manipulation jobs you have.
It’s critical to take into account factors like thread safety, performance consequences, and synchronization cost while choosing between StringBuilder and StringBuffer. You may maximize speed and ensure dependability across all of your Java apps by making an informed decision based on your unique requirements.