Java developers frequently stumble into the java.lang.UnsupportedClassVersionError while trying to execute a Java applet or application. When the Java Virtual Machine (JVM) comes across a class file that was built using a higher version of Java than what it supports, this error is produced. Usually, the error message says “Unsupported major.minor version,” which means that the Java version that was used to compile the class file is not supported by the JVM.
We will examine the reasons behind the UnsupportedClassVersionError, go over typical situations where it arises, and offer workable fixes for the problem in this extensive tutorial. Regardless of your level of Java development knowledge, this tutorial will assist you in comprehending and resolving this annoying problem.
UnsupportedClassVersionError Error Example
The UnsupportedClassVersionError error, which indicates that the class com. rollbar is displayed in the sample below. Although Java 8 (class file version 52) was used for execution, Java 17 (class file version 61) was used for the compilation of ExampleApp.
Exception in thread "main" java.lang.UnsupportedClassVersionError: com/rollbar/ExampleApp
has been compiled by a more recent version of the Java Runtime (class file version 61.0),
this version of the Java Runtime only recognizes class file versions up to 52.0
Java Version Numbers
Let’s quickly review the Java version numbers for reference. This will be useful if we have to get the correct Java version.
Use the following table to map the versions:
Major | Minor | Java platform version |
45 | 3 | 1.1 |
46 | 0 | 1.2 |
47 | 0 | 1.3 |
48 | 0 | 1.4 Example: 1.4.x |
49 | 0 | 1.5 Example: 1.5 |
50 | 0 | 1.6 |
51 | 0 | 1.7 |
52 | 0 | 1.8 |
Using a newer Java version
Updating the JRE on the computer running the code is the easiest way to solve the issue. The directory where the Java installation is now located and its version number may be found by running the commands echo %JAVA_HOME% and java -version within a terminal. Identifying the version that is being used when several JREs are installed on the same computer might be especially helpful with this. The UnsupportedClassVersionError problem can be resolved by downloading and changing the JAVA_HOME variable to refer to the more recent version of Java (such as Java 17) .
Fix via the Command Line
Let’s now talk about how to fix this mistake while using the command line to execute Java. We may either run our code on a more recent version of Java or build it for an earlier version, depending on the circumstances.
Our circumstances will determine the ultimate choice. Running our program using a more recent version of Java is likely the best option if we need to use a third-party library that has already been built at a higher level. It may be preferable to compile down to an earlier version if we’re packaging a program for distribution.
JAVA_HOME Environment Variable
First, let’s examine the configuration of our JAVA_HOME variable. This will provide information about the JDK that is being used when we execute javac via the command line:
echo %JAVA_HOME%
Output:
C:\Apps\Java\jdk8-x64
Running a New JRE
Let’s go back to our example and see if we can fix the problem by running it at a higher Java version. We may use the java command that comes with Java 11 JRE to run our code, assuming that it is located at C:\Apps\jdk-11.0.2.
C:\Apps\jdk-11.0.2\bin\java com.baeldung.MajorMinorApp
Output:
Hello World!
Compiling With an Older JDK
It is necessary to compile the code for the specific Java version that we want to be able to run while developing an application.
We may accomplish it in three different ways: by compiling our code with an earlier JDK; by using the -release option (JDK 9 and newer); or by utilizing the -bootclasspath, -source, and -target parameters of the javac command (JDK 8 and older).
First, let’s execute our code with an earlier JDK, just like we did with a newer JRE:
C:\Apps\Java\jdk1.8.0_31\bin\javac com/baeldung/MajorMinorApp.java
Even if you only use -source and -target, class files incompatible with earlier Java versions may still be created.
We may point -bootclasspath at the intended JRE’s rt.jar to guarantee compatibility:
javac -bootclasspath "C:\Apps\Java\jdk1.8.0_31\jre\lib\rt.jar" \
-source 1.8 -target 1.8 com/baeldung/MajorMinorApp.java
JDK 8 and below are the primary targets of the aforementioned. The -release argument was added to JDK 9 in place of the -source and -target parameters. Targets 6, 7, 8, 9, 10, and 11 are supported with the –release option.
Let’s aim for Java 8 by using -release:
javac --release 8 com/baeldung/MajorMinorApp.java
Now we can run our code on a Java 8 or higher JRE.
Working with IDEs
Configuration settings for JDK and JRE versions may be found in all major IDEs, and they can be adjusted down to the project level. Setting up a Java project is simple with the latest integrated development environments (IDEs). This is especially true for graphical user interfaces, which enable users to download and install subsequent versions immediately. To fix the aforementioned UnsupportedClassVersionError problem, the project may be rebuilt or recompiled, and a setting commonly referred to as “Project language level” or “Compiler compliance level” can be adjusted—all without ever having to exit the IDE. Below is an example of where to look for this setting in the JetBrains IDEs.
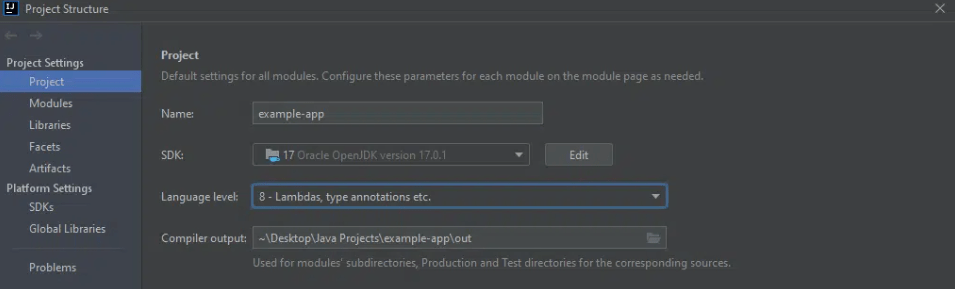
Maven Projects
It is feasible to regulate the Java version that is targeted by the compilation process while working with Maven projects, which is what most small and big corporate Java programs are. This is done using the Maven configuration, or Maven Project Object Model (POM) file. The following figure displays the pertinent parameters.
Although the source and destination versions can be controlled separately, it is best to set them to identical values because there is no assurance that the produced bytecode will work backward .
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
...
<properties>
<maven.compiler.source>17</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
...
</project>
Troubleshooting Tips
- To determine the version of the class file and the Java version that the JVM supports, carefully read the error message.
- For details on supported class file versions, consult your JDK or JRE’s documentation and release notes.
- Examine class files with diagnostic tools like “javap” to confirm version numbers.
- To handle dependencies and guarantee uniform compilation settings across projects, think about utilizing a build tool like Apache Maven or Gradle.
During the class linking process at the start of a program’s execution, a significant Java runtime fault known as the UnsupportedClassVersionError error might occur. This issue happens when you try to execute code on a platform with a JRE older than the one the code was created on. The code in question is often a fully functional pre-compiled program. To fix the UnsupportedClassVersionError problem, the target machine’s JRE must be updated. Alternatively, if backward compatibility is required, the program must be recompiled to target the earlier JRE. Although the same may be done without them, as this article has described, modern IDEs make this process easier with their built-in tools and customizable choices.