- Home
- /
- Programming
- /
- Page 14
Tutorial Category: Programming
Programming is the process of designing and writing computer programs, which are sets of instructions that tell a computer what to do. Programming involves several key concepts, including:
Algorithm: An algorithm is a step-by-step procedure for solving a problem. Programmers use algorithms to design solutions to specific problems, which are then translated into code.
Data structures: Data structures are ways of organizing and storing data in a computer program. Some common data structures include arrays, lists, and maps.
Control flow: Control flow refers to the order in which instructions are executed in a program. Control flow is controlled by conditional statements, loops, and functions.
Variables: Variables are used to store data in a program. They can hold different types of data, such as numbers, strings, and Boolean values.
Functions: Functions are reusable blocks of code that perform a specific task. Functions can take input parameters and return output values.
Object-oriented programming: Object-oriented programming is a programming paradigm that emphasizes the use of objects, which are instances of classes that encapsulate data and behavior.
Debugging: Debugging is the process of finding and fixing errors in a program. Programmers use various tools and techniques to identify and resolve bugs in their code.
Programming involves a combination of creativity, problem-solving skills, and attention to detail. Programmers must be able to break down complex problems into smaller, more manageable pieces, and design elegant solutions that are efficient, maintainable, and easy to understand.
Check if Value is NaN in Python
Python Print Flush Method
How to check if directory exists in Python
DataFrame Reorder Columns in Python
Python Advanced: Improve Your Python Programming Knowledge
Python Advanced: How to use Lambda Function with python Methods
Python Advanced: How to use Doctest and Unit Test in Python
Python Advanced: What is Test-Driven Development (TDD) with Python
Python Advanced: What is the Zip method in Python
Python Advanced: How to Utilize Memoization Technique in Python
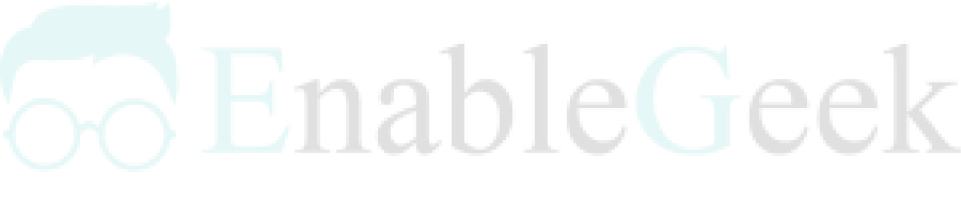
Check Our Ebook for This Online Course
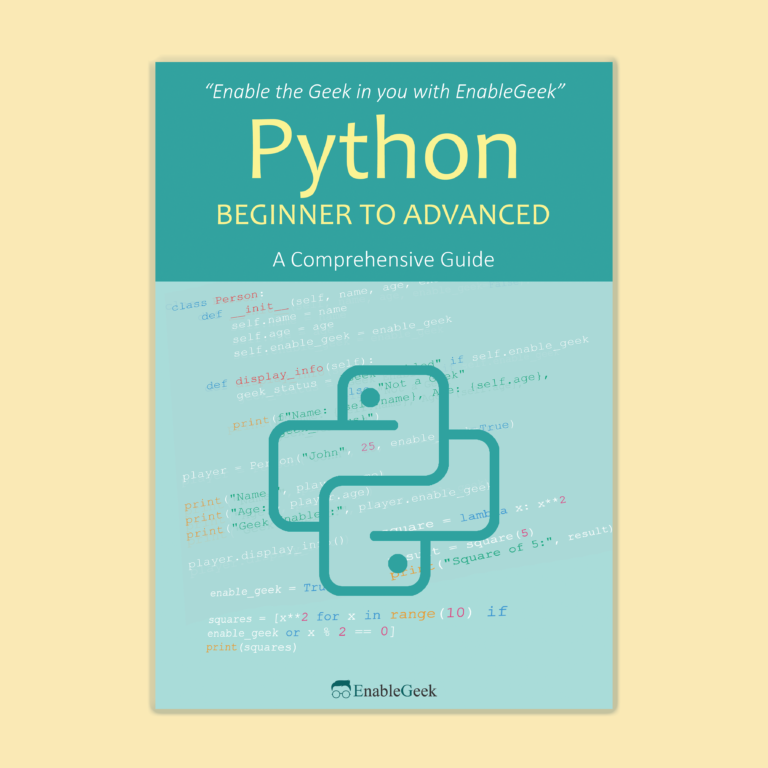
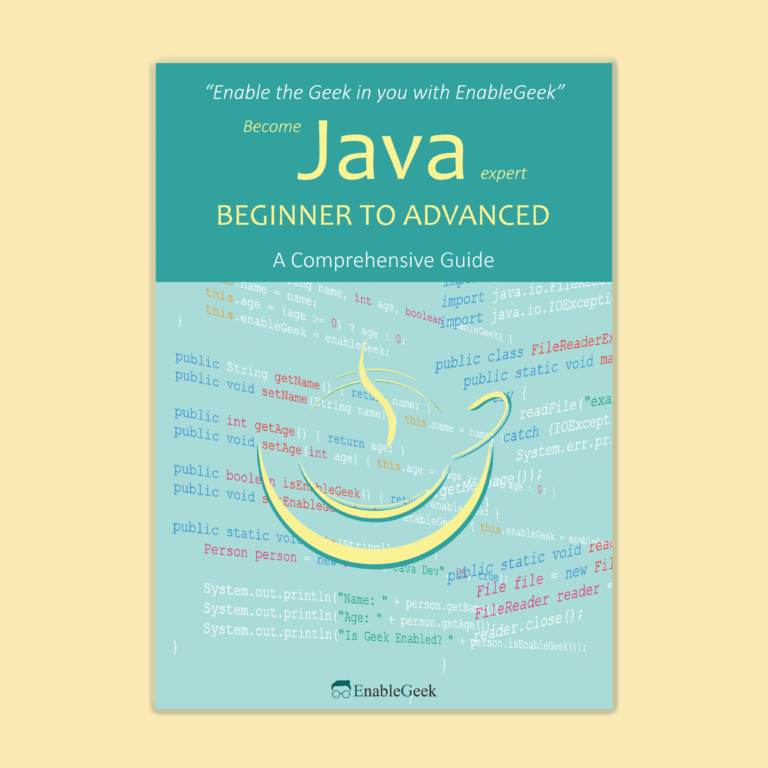
Advanced topics are covered in this ebook with many examples.